C++ class inheritance, key concept
What’s inherited
All base class members are inherited to derived class, except for:
- its constructors and its destructor
- its assignment operator members (operator=)
- its friends
Note: private members are inherited but not directly accessible by the derived class.
Inheritance access specifier
- public: mostly used. Allows the derived class to access public and protected members of the base class.
- protected: turns public members of the base class to potected.
- private: turns public and protected members of the base class to private.
Order of execution
- Constructor: First base class then derived class
- Destructor: First derived class then base class
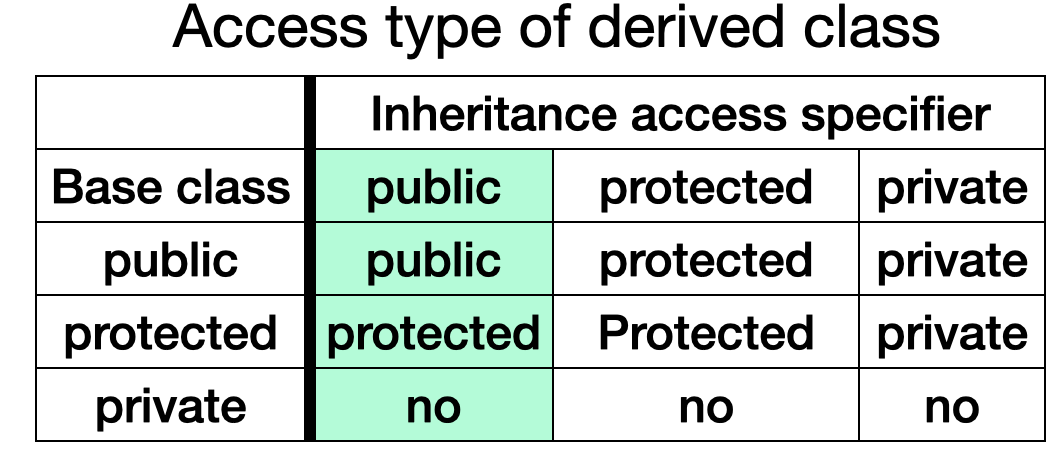
Code Example
Below is an example illustrating these relationships:
Explanation
Class Parent:
- Contains a private member privateMember and a public member publicMember.
- Defines a default constructor that initializes these members and prints a message.
- Defines a destructor that prints a message.
- Provides a public member function getPrivateMember() to access the private member.
Class Child1:
- Publicly inherits from Parent.
- The public inheritance allows Child1 to access the public members of Parent.
- Defines a constructor and destructor that print messages.
Class Child2:
- Privately inherits from Parent.
- The private inheritance means the inherited public members of Parent become private members of Child2.
- Defines member functions getParentPublicMember() and getParentPrivateMember() to access the inherited members.
- Defines a constructor and destructor that print messages.
Main Function:
- Creates objects of Child1 and Child2.
- Demonstrates access to public members and member functions of the parent class through child objects.
- Shows the order of constructor and destructor calls.
Conclusion
Understanding access specifiers and inheritance is crucial for designing robust and maintainable object-oriented programs in C++. Constructors and destructors help manage the lifecycle of objects, ensuring proper initialization and cleanup.